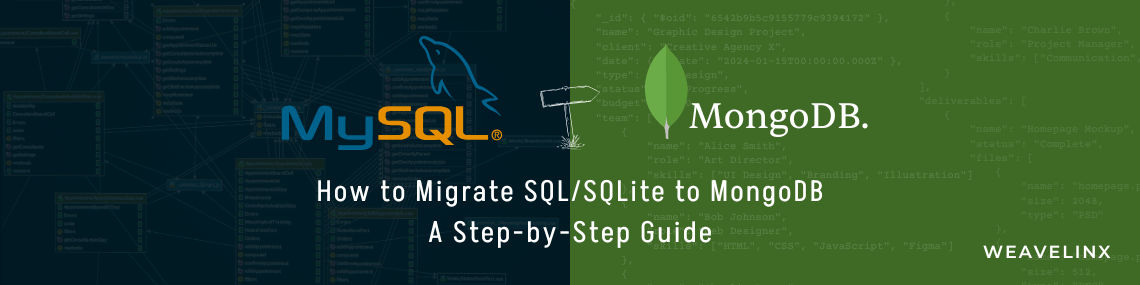
Table Of Content
- So, you’re thinking about switching…
- Relational vs Non-Relational – What’s the real difference?
- SQL vs SQLite vs MongoDB – A casual breakdown
- Why bother with MongoDB anyway?
- Step-by-step guide: Migrating your data
- Option 1: Using Tools (for the “just get it done” crowd)
- Option 2: Writing a Custom Script (for control freaks like us)
- Step 1: Start with the schema — understand your structure
- Step 2: Define where things go in MongoDB
- Step 3: Connect to MongoDB from your script
- Step 4: Write a little helper function to do the heavy lifting
- Step 5: Migrate the structure — your tables, collections, etc.
- Step 6: Migrate the actual data (the good stuff)
- Step 7: Done. Close it up and test like a mad scientist
- Migration Tips & FAQs
- Some things we wish we knew earlier
So, you’re thinking about switching from SQL or SQLite to MongoDB?
Maybe you’re building something that needs a bit more breathing room. Maybe you’re tired of wrestling with table joins every time you need nested data. Or maybe, you’re just curious what all the MongoDB hype is about. Whatever got you here, this guide’s for you.
We’re going to walk through the difference between relational and non-relational databases (without putting you to sleep), talk about what MongoDB does differently, and then—yep—actually move your data over. Whether you like tools or prefer to roll up your sleeves with a script, we’ve got both.
Relational vs Non-Relational: What’s the Real Deal?
If you’ve worked with SQL, you already know the relational world. Think tables. Rows. Columns. Everything has its place. It’s neat, tidy, and rigid. You define your schema up front, and your data better follow the rules.
Now MongoDB? It’s like the cool cousin who doesn’t care if your data brings weird fields to the party. Instead of tables, Mongo uses collections. Instead of rows, you’ve got documents. It’s less strict, more flexible.
Imagine storing a customer and all their orders in one single document — no JOINs needed. That’s what MongoDB is about.
Some Quick Examples
- Relational: MySQL, PostgreSQL, SQLite
- Non-relational (NoSQL): MongoDB, Couchbase, Cassandra
SQL vs SQLite vs MongoDB (Let’s Clear This Up)
Feature | SQL (e.g., MySQL/Postgres) | SQLite | MongoDB |
---|---|---|---|
What it is | A full-blown relational database system | A lightweight, file-based version of SQL | A NoSQL, document-based database |
Storage | Stores data on a server with multiple files and processes | Everything’s packed into a single .sqlite file | Stores JSON-like documents in collections |
Setup | Needs a server, user, password, ports… you know, the usual | Just give it a file and you’re good to go | Needs a MongoDB server running, but setup’s pretty simple |
Schema | Strict. You define tables, columns, types ahead of time | Same as SQL — rigid structure | Flexible. No need to pre-define structure unless you want to |
Best For | Big applications, enterprise stuff, multi-user systems | Local apps, mobile projects, small tools | Modern apps, rapid development, APIs, flexible data needs |
Relationships | Foreign keys, JOINs, all the classic tools | Supports foreign keys, but people rarely use them | You embed or reference — no joins, just clever structuring |
Scaling | Vertically (add more power to the same machine) | Doesn’t really scale — it’s for local use | Horizontally (add more servers to the cluster) |
Performance | Fast with the right tuning, but can get complex | Fast for small apps, but not built for high traffic | Scales well with big data, but structure planning matters |
Language | Good ol’ SQL (SELECT * FROM users ) | Same syntax as SQL | Different syntax (db.users.find({}) ) but super readable |
Why Bother with MongoDB?
Here’s the short version of why people switch:
- It scales sideways (add more servers, not just more RAM).
- No schema rigidity — add fields whenever you want.
- Related data can live together in the same place.
- It plays nice with modern web apps and JSON-heavy APIs.
- Fewer JOINs. Fewer headaches.
For fast-moving teams, startups, or apps with constantly changing data, MongoDB can feel like a breath of fresh air.
Step-by-Step Guide: Moving from SQL or SQLite to MongoDB
Alright. Time to migrate. You’ve got two ways to go: use tools (fast and easy), or write a custom script (a bit more work, but total control). Let’s check both.
Option 1: Using Tools (Good for Small to Medium Projects)
If you just want to get data across without much fuss, tools are your friend:
- mongoimport: Great for importing from CSV/JSON.
- MongoDB Compass: Has a simple import wizard.
- MongoDB Relational Migrator: Converts schemas and code, works for major SQL databases.
- Studio 3T: Commercial, but super visual and supports complex mapping.
- sqlitemongo: A handy CLI tool just for SQLite to MongoDB.
These tools are handy, but they don’t give you full control over how your data is transformed. If you need to merge tables, restructure relationships, or embed data, you’ll want the scripting route.
Option 2: Writing a Custom Script (For the Brave and the Curious)
This is where you turn into the boss of your data. You get to decide exactly how things are structured in MongoDB.
Here’s a working example using Node.js to migrate from SQLite. The logic is simple: grab the data from SQLite, shape it how MongoDB likes it, and push it over.
Note: If you’re using MySQL or PostgreSQL instead of SQLite, you just need to swap out the database connection code. The mapping and transformation logic stays the same.
Step 1: Start with the usual suspects
const { MongoClient, ObjectId } = require("mongodb");
const sqlite3 = require("sqlite3").verbose();
Step 2: Define where your stuff is
const sqliteDbPath = "database.sqlite";
const mongoUri = "mongodb://localhost:27017";
const mongoDbName = "my-user";
Just your database file and Mongo connection string. Nothing fancy.
Step 3: Connect to MongoDB and SQLite
const client = new MongoClient(mongoUri, { useUnifiedTopology: true });
await client.connect();
const mongoDb = client.db(mongoDbName);
const studentsCollection = mongoDb.collection("students");
const studentMarkCollection = mongoDb.collection("students_marks");
We get into MongoDB, pick our database, and prep the collections we’ll be inserting into.
const sqliteDb = new sqlite3.Database(sqliteDbPath, sqlite3.OPEN_READONLY);
This line hooks us up with our SQLite file. If you’re using MySQL, you’d do something like:
const mysql = require('mysql2/promise');
const connection = await mysql.createConnection({ /* your config */ });
Step 4: Write a little helper for clean number conversions
const toNumber = (value) => (value !== null && !isNaN(value) ? parseFloat(value) : 0);
Because not all your data will be perfect. Some cleanup is just good hygiene.
Step 5: Migrate the students table
const sqliteAll = (query, params = []) =>
new Promise((resolve, reject) => {
sqliteDb.all(query, params, (err, rows) => {
if (err) reject(err);
else resolve(rows);
});
});
const students = await sqliteAll("SELECT * FROM students");
const studIdMap = {};
const formattedStudents = students.map((student) => {
const newStudId = new ObjectId();
studIdMap[student.studId] = newStudId;
return {
_id: newStudId,
roll_no: student.rollNo,
name: student.name,
};
});
await studentsCollection.insertMany(formattedStudents);
Step 6: Migrate marks and link to students
const studentMarks = await sqliteAll("SELECT * FROM students_marks");
const formattedStudentsMarks = studentMarks
.map((line) => {
const mappedStudId = studIdMap[line.studId];
if (!mappedStudId) return null;
return {
_id: new ObjectId(),
stud_id: mappedStudId,
mark: toNumber(line.mark),
};
})
.filter(Boolean);
await studentMarkCollection.insertMany(formattedStudentsMarks);
Step 7: Done. Close it up
sqliteDb.close();
await client.close();
console.log("Migration complete!");
You’ve officially moved your data from SQLite to MongoDB. Go get a snack, you earned it.
Migration Tips & FAQs
- Start with a test migration. Don’t just go all in on production data.
- Embed where it makes sense, reference when it doesn’t.
- Check document size limits in MongoDB (16MB max per doc).
- Create indexes early for fast reads, or things might feel slower than they should.
- You can always clean up data during the move. Take advantage of the chance to fix weird formats, duplicates, etc.
Common questions people ask:
Q: Can MongoDB handle my foreign keys?
Q: Do I have to rewrite my whole app to work with MongoDB?
A: If your app uses a lot of SQL queries, yeah, you’ll need to rewrite those. But hey, MongoDB’s syntax is not that bad once you get used to it.
Q: How do I keep two databases in sync during a slow migration?
A: You’ll want something like change data capture (CDC) or just build a “dual write” logic temporarily.
That’s a wrap for this section. You’ve got tools if you’re in a hurry, and scripting if you want control. Either way, MongoDB’s door is wide open, and your data is ready for a new home.
Let me know if you’d like this converted into a downloadable blog post or newsletter format. Happy to help!